State-of-the-Art Shitcode Principles
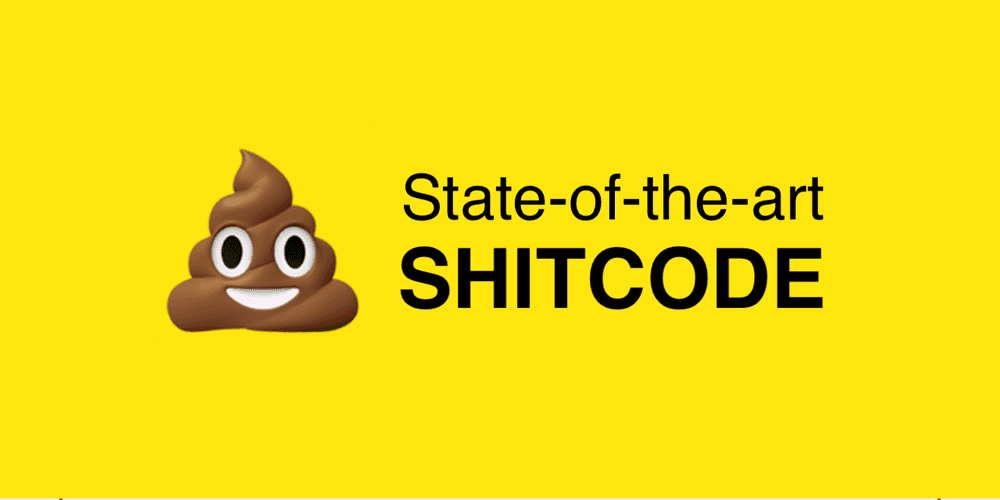
This a list of state-of-the-art shitcode principles your project should follow.
Get Your Badge
If your repository follows the state-of-the-art shitcode principles you may use the following "state-of-the-art shitcode" badge:
[](https://github.com/trekhleb/state-of-the-art-shitcode)
The Principles
π© Name variables in a way as if your code was already obfuscated
Fewer keystrokes, more time for you.
Good ππ»
let a = 42;
Bad ππ»
let age = 42;
π© Mix variable/functions naming style
Celebrate the difference.
Good ππ»
let wWidth = 640;
let w_height = 480;
Bad ππ»
let windowWidth = 640;
let windowHeight = 480;
π© Never write comments
No one is going to read your code anyway.
Good ππ»
const cdr = 700;
Bad ππ»
More often comments should contain some 'why' and not some 'what'. If the 'what' is not clear in the code, the code is probably too messy.
// The number of 700ms has been calculated empirically based on UX A/B test results.
// @see: <link to experiment or to related JIRA task or to something that explains number 700 in details>
const callbackDebounceRate = 700;
π© Always write comments in your native language
If you violated the "No comments" principle then at least try to write comments in a language that is different from the language you used to write the code. If your native language is English you may violate this principle.
Good ππ»
// ΠΠ°ΠΊΡΠΈΠ²Π°ΡΠΌΠΎ ΠΌΠΎΠ΄Π°Π»ΡΠ½Π΅ Π²ΡΠΊΠΎΠ½Π΅ΡΠΊΠΎ ΠΏΡΠΈ Π²ΠΈΠ½ΠΈΠΊΠ½Π΅Π½Π½Ρ ΠΏΠΎΠΌΠΈΠ»ΠΊΠΈ.
toggleModal(false);
Bad ππ»
// Hide modal window on error.
toggleModal(false);
π© Try to mix formatting style as much as possible
Celebrate the difference.
Good ππ»
let i = ['tomato', 'onion', 'mushrooms'];
let d = [ "ketchup", "mayonnaise" ];
Bad ππ»
let ingredients = ['tomato', 'onion', 'mushrooms'];
let dressings = ['ketchup', 'mayonnaise'];
π© Put as much code as possible into one line
Good ππ»
document.location.search.replace(/(^\?)/,'').split('&').reduce(function(o,n){n=n.split('=');o[n[0]]=n[1];return o},{})
Bad ππ»
document.location.search
.replace(/(^\?)/, '')
.split('&')
.reduce((searchParams, keyValuePair) => {
keyValuePair = keyValuePair.split('=');
searchParams[keyValuePair[0]] = keyValuePair[1];
return searchParams;
},
{}
)
π© Fail silently
Whenever you catch an error it is not necessary for anyone to know about it. No logs, no error modals, chill.
Good ππ»
try {
// Something unpredictable.
} catch (error) {
// tss... π€«
}
Bad ππ»
try {
// Something unpredictable.
} catch (error) {
setErrorMessage(error.message);
// and/or
logError(error);
}
π© Use global variables extensively
Globalization principle.
Good ππ»
let x = 5;
function square() {
x = x ** 2;
}
square(); // Now x is 25.
Bad ππ»
let x = 5;
function square(num) {
return num ** 2;
}
x = square(x); // Now x is 25.
π© Create variables that you're not going to use.
Just in case.
Good ππ»
function sum(a, b, c) {
const timeout = 1300;
const result = a + b;
return a + b;
}
Bad ππ»
function sum(a, b) {
return a + b;
}
π© Don't specify types and/or don't do type checks if language allows you to do so.
Good ππ»
function sum(a, b) {
return a + b;
}
// Having untyped fun here.
const guessWhat = sum([], {}); // -> "[object Object]"
const guessWhatAgain = sum({}, []); // -> 0
Bad ππ»
function sum(a: number, b: number): ?number {
// Covering the case when we don't do transpilation and/or Flow type checks in JS.
if (typeof a !== 'number' && typeof b !== 'number') {
return undefined;
}
return a + b;
}
// This one should fail during the transpilation/compilation.
const guessWhat = sum([], {}); // -> undefined
π© You need to have an unreachable piece of code
This is your "Plan B".
Good ππ»
function square(num) {
if (typeof num === 'undefined') {
return undefined;
}
else {
return num ** 2;
}
return null; // This is my "Plan B".
}
Bad ππ»
function square(num) {
if (typeof num === 'undefined') {
return undefined;
}
return num ** 2;
}
π© Triangle principle
Be like a bird - nest, nest, nest.
Good ππ»
function someFunction() {
if (condition1) {
if (condition2) {
asyncFunction(params, (result) => {
if (result) {
for (;;) {
if (condition3) {
}
}
}
})
}
}
}
Bad ππ»
async function someFunction() {
if (!condition1 || !condition2) {
return;
}
const result = await asyncFunction(params);
if (!result) {
return;
}
for (;;) {
if (condition3) {
}
}
}
π© Mess with indentations
Avoid indentations since they make complex code take up more space in the editor. If you're not feeling like avoiding them then just mess with them.
Good ππ»
const fruits = ['apple',
'orange', 'grape', 'pineapple'];
const toppings = ['syrup', 'cream',
'jam',
'chocolate'];
const desserts = [];
fruits.forEach(fruit => {
toppings.forEach(topping => {
desserts.push([
fruit,topping]);
});})
Bad ππ»
const fruits = ['apple', 'orange', 'grape', 'pineapple'];
const toppings = ['syrup', 'cream', 'jam', 'chocolate'];
const desserts = [];
fruits.forEach(fruit => {
toppings.forEach(topping => {
desserts.push([fruit, topping]);
});
})
π© Do not lock your dependencies
Update your dependencies on each new installation in uncontrolled way. Why stick to the past, let's use the cutting edge libraries versions.
Good ππ»
$ ls -la
package.json
Bad ππ»
$ ls -la
package.json
package-lock.json
π© Always name your boolean value a flag
Leave the space for your colleagues to think what the boolean value means.
Good ππ»
let flag = true;
Bad ππ»
let isDone = false;
let isEmpty = false;
π© Long-read functions are better than short ones.
Don't divide a program logic into readable pieces. What if your IDE's search breaks and you will not be able to find the necessary file or function?
- 10000 lines of code in one file is OK.
- 1000 lines of a function body is OK.
- Dealing with many services (3rd party and internal, also, there are some helpers, database hand-written ORM and jQuery slider) in one
service.js
? It's OK.
π© Avoid covering your code with tests
This is a duplicate and unnecessary amount of work.
π© As hard as you can try to avoid code linters
Write code as you want, especially if there is more than one developer in a team. This is a "freedom" principle.
π© Start your project without a README file.
Keep it that way for the time being.
π© You need to have unnecessary code
Don't delete the code your app doesn't use. At most, comment it.
Subscribe to the Newsletter
Get my latest posts and project updates by email